Numeric scale mode is the default scale mode of the PrimaryY and SecondaryY chart axes (for details, see StandardAxis Enumeration). When operating in this mode, the axes are scaled to fit the data contained in the data series of the series for the respective chart dimension.
The properties of the numeric scale are controlled through an instance of the AxisScaleNumeric class, which is accessible through the NumericScale property of the Axis class. The AxisScaleNumeric class is a direct descendant of the AxisScaleValue class and inherits all its functionality. It also implements control over the following aspects of a numeric scale.
Controlling Major Tick Generation in Numeric Scale Mode
The user can control the mode in which the major ticks are generated using the MajorTickMode property, which is of type MajorTickModeNumeric and can accept the following values (for details, see MajorTickModeNumeric Enumeration):
Manual
Auto
CustomStep
IrregularSteps
The following is a description of these modes:
- Manual: When operating in this mode, the scale does not produce any ticks. It is up to the user to specify values on which major ticks should be displayed. The following code will display ticks on values 10, 15, and 25 of the PrimaryY axis:
VB.NET |
|
Dim axis As Axis = Chart.Axis(StandardAxis.PrimaryY) axis.ScaleMode = AxisScaleMode.Numeric axis.NumericScale.MajorTickMode = MajorTickModeNumeric.Manual axis.MajorTicks.Clear() axis.MajorTicks.Add(10) axis.MajorTicks.Add(15) axis.MajorTicks.Add(25)
|
C# |
|
Axis axis = Chart.Axis(StandardAxis.PrimaryY); axis.ScaleMode = AxisScaleMode.Numeric; axis.NumericScale.MajorTickMode = MajorTickModeNumeric.Manual; axis.MajorTicks.Clear(); axis.MajorTicks.Add(10); axis.MajorTicks.Add(15); axis.MajorTicks.Add(25);
|
- Auto: Automatic mode produces major ticks at equal intervals. The step is automatically determined. In this mode the user can control the density of the automatically generated major ticks with the MaxTickCount property (inherited from AxisScaleValue). The following code instructs the PrimaryY axis to operate in Auto mode and generate no more than 10 major ticks, regardless of the data scaled on it.
VB.NET |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.Auto axis.NumericScale.MaxTickCount = 10
|
C# |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.Auto; axis.NumericScale.MaxTickCount = 10;
|
- Custom Step: Custom step mode produces automatic major ticks at equal intervals with user-specified step. The step is controlled by the CustomStep property. The following code will force the numeric scale to produce major ticks with a step of 20:
VB.NET |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.CustomStep axis.NumericScale.CustomStep = 20
|
C# |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.CustomStep; axis.NumericScale.CustomStep = 20;
|
- Irregular Steps: In this scaling mode, the scale produces automatic major ticks at irregular intervals. The user specifies an array of steps, through which the scale iterates. The following code will generate major ticks at irregular user-defined intervals:
VB.NET |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.IrregularSteps axis.NumericScale.CustomSteps.Clear() axis.NumericScale.CustomSteps.Add(10) axis.NumericScale.CustomSteps.Add(20) axis.NumericScale.CustomSteps.Add(15)
|
C# |
|
axis.NumericScale.MajorTickMode = MajorTickModeNumeric.IrregularSteps; axis.NumericScale.CustomSteps.Clear(); axis.NumericScale.CustomSteps.Add(10); axis.NumericScale.CustomSteps.Add(20); axis.NumericScale.CustomSteps.Add(15);
|
Demonstration of the Numeric Major Tick Modes |
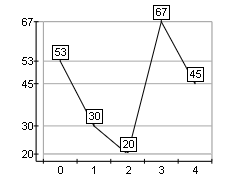 |
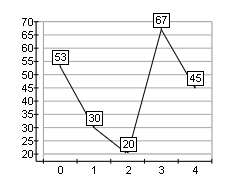 |
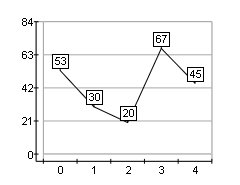 |
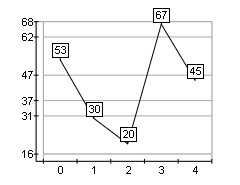 |
Controlling Scale Origin in Numeric Scale Mode
The scale origin is a value, which if contained in the axis scale range is always displayed with a tick mark. By default the numeric scale uses 0 as its origin.
Two properties of the numeric scale give you control over the scale origin. The first one, UseOrigin, specifies whether an origin should be used. If you set this property to false, ticks will begin at the min value displayed by the axis. The second one, Origin, specifies the actual origin value and is by default set to 0. It is valid only if the UseOrigin property is set to true. The following code displays the axis with the origin at 2.5:
VB.NET |
|
axis.NumericScale.UseOrigin = True axis.NumericScale.Origin = 2.5
|
C# |
|
axis.NumericScale.UseOrigin = true; axis.NumericScale.Origin = 2.5; |
Related Examples
Windows Forms: Axes\Scaling\Numeric Scale
See Also
Axis Scales | Axis | AxisScale | AxisScaleValue | AxisScaleNumeric